Adding a tablet pdf annotation component to your web application
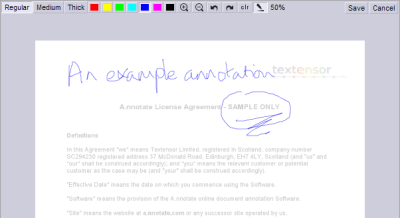
This page explains how to use A.nnotate to add the capability for handwritten annotation to your web application. You can display a new window open on a particular page for hand signing with a tablet pen device. The API allows you to extract the points on the line, with timestamps at each point (so for example you can compute pen velocity). If Silverlight is installed and a pressure-sensitive tablet is used, then pen pressure information is also collected. The system use HTML Canvas, VML, or Silverlight according to what is available.
- 1. freehand.php - Showing an document freehand annotation component in an iframe
- 2. freethumb.php - Generating an annotated thumbnail image when a user is logged in
- 3. apiFreehandThumb.php - Generating an annotated thumbnail image via the server API
1. Showing a document annotation component in an iframe or popup window
You can use freehand.php to display a freehand document annotation component in an iframe or a new window. You should log the user in first (e.g. with the loginAs api call), then set the src attribute of the iframe or popup window to:
http://yoursite.com/annotate/php/freehand.php? &d=2008-01-02 # The document upload date &c=abc123 # the document code &p=1 # the page number &ic=0 # (optional) hide the ink color chooser &ink=ff0000 # (optional) set the initial ink color (hex RGB) &tb=0 # (optional) hide the toolbar &as=0 # (optional) switch auto-save off (1 to switch it on)
An example showing how to embed a freehand component is included in local A.nnotate installations (since August 2010): php/freehandexample.php. This also demonstrates how you can add JavaScript callbacks to your frame to be notified when a new drawing has been added. If auto-save it switched on, then new lines will be automatically saved after a second or so of inactivity.
The freehand routine automatically clusters drawings into separate fragments; each fragment has a bounding box and a collection of nearby lines. This is to make user selection easier; if a user makes small notes in each corner of a page, these will be saved as four separate fragments, each with a small bounding box (rather than a single drawing with full page bounding box). In this case, when the user presses the 'save' button, the noteSaved(dat) callback will be called four times, and then the saveComplete() function will be called to notify that all fragments have been saved.
// If you provide these functions in the parent window then they // will be called when the user presses the 'Save' or 'Cancel' // button. There is a worked example in freehandexample.php: function noteSaved(dat) { msg("Freehand fragment saved " + dat); /* Example drawing object: { boundingBox: [ 100, 100, 30, 40 ], // x, y, w, h drawing: [ // array of lines { color: '#000000', // line color width: 1, // line width np: 26, // number of points time: 20, // time offset of point 1 (ms) xpts: [ 1,1,2, ... ], // array of x coords (rel to bounding box x,y) ypts: [ 1,1,2, ... ], // array of y coords (rel to bounding box x,y) times: [ 1,1,2, ... ], // array of times (ms) pressures: [ 50, 75, 75 ... ], // array of pressures [1-100] }, { // ... line 2 etc } ] } */ } function saveComplete() { msg("All fragments now saved"); } function noteCancelled() { msg("Annotation cancelled"); }
For more on the format of the drawing field above, see the API reference on freehand notes.
2. Generating an annotated thumbnail image when the user is logged in
To make a thumbnail of the page including the hand drawn annotations, you can call the freethumb.php utility with the given width and height in pixels:
http://yoursite.com/annotate/php/freethumb.php? &d=2008-01-02 # The document upload date &c=abc123 # the document code &p=1 # the page number &w=300 # width in pixels &h=200 # height in pixels &fmt=txt # Return format: # txt - returns a plain text url # src - redirects to the image # html - a basic html wrapper (for testing) &nocache=0 # (optional) set to 1 to force regeneration of the image.
The thumbnail will be a png image scaled to fit inside a rectangle of width w and height h. For the return format, the fmt argument can be "txt" (for a url) or "src" (so the freethumb.php call can be used as the source of an image). If there is a problem then the call will return plain text: "ERR {error message}".
3. Generating an annotated thumbnail image using the API
You can also get a link to a freehand thumbnail using a signed API call from your server:
http://yoursite.com/annotate/php/apiFreehandThumb.php? api-user=joe@example.com # The admin user for the account &api-requesttime=123456 # the Unix timestamp (GMT) &api-annotateuser=jill@example.com # The user's account &api-auth=xyz1234543983jeflgnwefgdgd # The signed hash code. &d=2008-01-02 # The document upload date &c=abc123 # the document code &p=1 # the page number &w=300 # width in pixels &h=200 # height in pixels &fmt=txt # Return link to image in format: # txt - plain text url # src - redirect to the image
The return value is OK {url_of_thumbnail} {file_modification_time}, or ERR {message}. If fmt=src, then the call will redirect to the image (so the signed api call can be used as an image source.) See the main api reference for more details on making api calls.